Are you looking to add a personal touch to your WooCommerce store? One often overlooked area is the customization of order emails. These emails are a crucial part of your customer communication strategy, providing essential information about their purchases and reinforcing your brand identity.
As you know WooCommerce platform provides many customization options, including the ability to tailor your order confirmation, processing, and shipping emails to align with your brand’s identity and provide relevant information to your customers.
In this guide, we’ll delve into how to customize WooCommerce order emails, covering everything from the importance of customization to practical code examples using hooks.
Why Should You Customize Your Emails?
The first question that might come to mind is, why bother customizing WooCommerce order emails? So, before we dive into the technical details, let’s talk about why customizing your WooCommerce order emails is so important.
The answer lies in enhancing customer engagement, reinforcing your brand identity, and providing a personalized experience to your shoppers.
Generic, impersonal emails can make your customers feel disconnected from your brand. By customizing your emails, you can create a more engaging and great experience for your customers.
Personalized emails are also more likely to be opened and read, leading to increased customer satisfaction and loyalty. Additionally, customizing your emails allows you to reinforce your brand identity and create a consistent experience across all touchpoints.
Customize Order Emails by WooCommerce Settings
Customizing order emails in WooCommerce through settings is a straightforward process that allows you to modify the design and content of your emails without delving into code.
Here are the steps to customize order emails using WooCommerce settings.
- Access WooCommerce Settings: Log in to your WordPress dashboard and navigate to WooCommerce > Settings.
- Navigate to the Emails Tab: Click on the “Emails” tab at the top of the settings page. This will take you to the email settings section.
- Select the Email Template to Customize: Scroll down to view the list of available email templates. WooCommerce provides templates for various order-related emails such as New Order, Processing Order, Completed Order, etc.
- Customize Email Header, Footer, and Color: The email customizer allows you to modify the header and footer of the email template. You can add your logo, change the colors, and include any additional information or branding elements.
- Click on the Email Template to Customize: Choose the email template you want to customize from the list. For example, if you want to customize the email sent to customers when a new order is placed, click on the “New Order” template.
- Edit Email Heading and Body Content: Next, you’ll find the heading and email body content editor. Here, you can modify the text and formatting of the email message. You can also use placeholders to dynamically insert order-related information such as order number, order total, and customer details.
- Save Changes: Once you’re satisfied with your customizations, click the “Save changes” button to save your settings.
- Repeat for Other Email Templates (Optional): If you want to customize additional email templates, such as the processing order or completed order emails, repeat the above steps for each template.
- Test Your Emails: Before making your customizations live, it’s essential to test them to ensure everything looks and functions as intended. Place a test order on your website and verify that you receive the customized email with the correct information and formatting.
By following these steps, you can easily customize order emails in WooCommerce using the built-in settings without requiring any coding knowledge.
Customize WooCommerce Order Emails By Using Hooks
Hooks play a great role in customizing WooCommerce email templates. Hooks give you the flexibility to inject custom code at various points throughout the email generation process.
See the following image for a visual reference of WooCommerce email hooks.
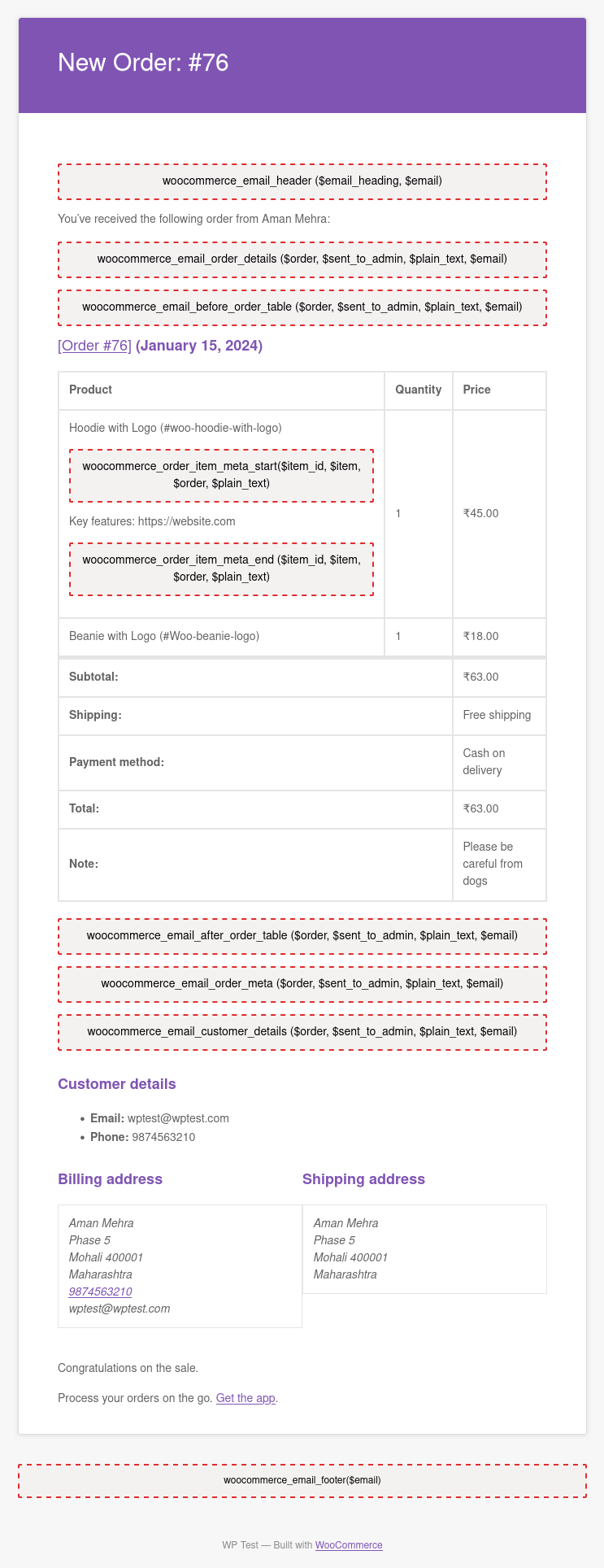
Let’s delve into some hooks to customize the WooCommerce order emails and explore how they can be utilized to tailor your email notifications effectively.
woocommerce_email_header( $email_heading, $email )
This hook allows you to customize the email header, typically used to modify the heading or title of the email. You can leverage this hook to provide a personalized touch to your email notifications based on specific criteria.
For example, you might want to change the header of the completed order email to express gratitude, as shown in the following code example.
function custom_email_header($email_heading, $email) { // Check if the email is for completed orders if ($email->id === 'customer_completed_order') { // Modify the email header $email_heading = 'Thank you for your purchase!'; } // Return the modified email header return $email_heading; } add_filter('woocommerce_email_header', 'custom_email_header', 10, 2);
In this example, we’re checking if the email ID matches ‘customer_completed_order‘, which corresponds to the completed order notification. If it does, we’re updating the email heading to ‘Thank you for your purchase!’. This allows you to customize the email header specifically for completed order emails.
woocommerce_email_order_details( $order, $sent_to_admin, $plain_text, $email )
With this hook, you can customize the order details section of the email. This is where you can include relevant information such as the order summary, billing and shipping addresses, and any additional details you want to communicate to the customer.
Whether you’re adding custom fields or modifying the layout, this hook offers ample opportunities for tailoring the order details to meet your specific requirements.
function custom_email_order_details($order, $sent_to_admin, $plain_text, $email) { // Append custom text to order details $order_details = $email->format_order_details($order, $sent_to_admin, $plain_text); $custom_text = 'Thank you for shopping with us!'; $order_details .= "\n\n" . $custom_text; // Return modified order details return $order_details; } add_filter('woocommerce_email_order_details', 'custom_email_order_details', 10, 4);
This code adds a custom message (“Thank you for shopping with us!”) to the end of the order details section in the email.
woocommerce_email_before_order_table( $order, $sent_to_admin, $plain_text, $email )
The woocommerce_email_before_order_table
hook allows you to inject content before the order table in the email. This can be useful for including introductory messages, promotional offers, or any other information you want to highlight at the beginning of the email.
By strategically placing content using this hook, you can capture the recipient’s attention and enhance the overall effectiveness of your email communication.
Here’s an example of adding a promotional banner before the order table:
function custom_email_before_order_table($order, $sent_to_admin, $plain_text, $email) { // Add promotional banner before order table $promo_banner = '<img src="https://example.com/promo-banner.jpg" alt="Promotional Banner">'; return $promo_banner; } add_filter('woocommerce_email_before_order_table', 'custom_email_before_order_table', 10, 4);
This code inserts an image banner before the order table in the email. You can insert any other content like promotional messages, affiliate links, warning text, etc.
woocommerce_order_item_meta_start( $item_id, $item, $order, $plain_text )
This hook is specifically designed for customizing the order items meta, such as product details, quantity, and pricing.
You can use this hook to add or modify meta-information for each item in the order, providing additional context or relevant details that enhance the customer’s understanding of their purchase.
function custom_order_item_meta_start($item_id, $item, $order, $plain_text) { // Add custom content at the start of order item meta echo '<p>Custom Item Meta Start</p>'; } add_action('woocommerce_order_item_meta_start', 'custom_order_item_meta_start', 10, 4);
The above code will show the custom data before the item’s meta. You can make any functionality or logic or any static text and insert it before the order’s item metadata.
woocommerce_order_item_meta_end( $item_id, $item, $order, $plain_text )
Similar to the woocommerce_order_item_meta_start
hook, woocommerce_order_item_meta_end
allows you to customize the order item meta, but this time at the end of each item’s details.
This can be useful for appending additional information or adding closing remarks to each order item, further enriching the content of your email notifications.
function custom_order_item_meta_end($item_id, $item, $order, $plain_text) { // Add custom content at the end of order item meta echo '<p>Custom Item Meta End</p>'; } add_action('woocommerce_order_item_meta_end', 'custom_order_item_meta_end', 10, 4);
This code displays at the end of the order’s item meta and you can insert any dynamic data or static text.
woocommerce_email_after_order_table( $order, $sent_to_admin, $plain_text, $email )
This hook enables you to inject content after the order table in the email. Whether you want to include additional instructions, cross-sell recommendations, or social media links, this hook provides a convenient way to extend the email’s content beyond the standard order details, enhancing its value and relevance to the recipient.
function custom_email_after_order_table($order, $sent_to_admin, $plain_text, $email) { $additional_content = '<p>Thank you for shopping with us!</p>'; echo $additional_content; } add_action('woocommerce_email_after_order_table', 'custom_email_after_order_table', 10, 4);
In this code, we are adding additional content after the order table to thank message to customers for shopping with us.
woocommerce_email_order_meta( $order, $sent_to_admin, $plain_text, $email )
The woocommerce_email_order_meta
hook allows you to add custom metadata to the email, which is typically displayed after the order details.
This can include information such as order notes, payment method details, or any other relevant data that complements the order information and provides context to the recipient.
function custom_email_order_meta($order, $sent_to_admin, $plain_text, $email) { // Add custom metadata to the email echo '<p>Custom Order Meta: Your additional order meta information here</p>'; } add_action('woocommerce_email_order_meta', 'custom_email_order_meta', 10, 4);
You will see the custom metadata after the order detail by using the above code hook.
woocommerce_email_customer_details( $order, $sent_to_admin, $plain_text, $email )
This hook is used to customize the customer details section of the email, including the billing and shipping addresses, as well as any other customer-specific information.
By leveraging this hook, you can tailor the content and layout of the customer details to match your brand’s aesthetic and provide a seamless experience for the recipient.
function custom_email_customer_details($order, $sent_to_admin, $plain_text, $email) { // Add custom content to the customer details section echo '<p>Customer Details: Your custom content here</p>'; } add_action('woocommerce_email_customer_details', 'custom_email_customer_details', 10, 4);
This code will insert the custom information into the Customer Detail section of the WooCommerce order email.
Also Read: 10 Ways to Get User ID in WordPress
Finally, the woocommerce_email_footer
hook allows you to customize the email footer, which typically includes essential information such as contact details, unsubscribe links, and legal disclaimers.
This hook provides a convenient way to add branding elements, social media icons, or other relevant content to the email footer, ensuring consistency and professionalism across your email communications.
function custom_email_footer($email) { // Add custom content to the email footer echo '<p>Email Footer: Your custom footer content here</p>'; } add_action('woocommerce_email_footer', 'custom_email_footer');
If you want to add your custom content like social icons, branding, quick links, etc, then you the above code.
These examples demonstrate how you can use each hook to customize different aspects of WooCommerce order emails, from headers and order details to item metadata and footers.
By mastering these hooks and understanding how to use them effectively, you can unlock endless possibilities to customize WooCommerce order emails and deliver tailored, engaging experiences to your customers.
Customize WooCommerce Email Template by Overriding
Now, let’s get into customizing WooCommerce email templates. One of the most straightforward approaches is to modify the existing templates provided by WooCommerce.
You can access these visual interface of templates via the WordPress admin panel under WooCommerce > Settings > Emails. Here, you’ll find a list of email notifications along with options to customize their subject, heading, content, and footer.
By leveraging the built-in editor, you can customize the HTML and CSS of each template to match your brand’s aesthetic and messaging.
But if you want to add or customize the part of the email dynamically then you can modify the email template files in the WooCommerce plugin. Every email type has its own template file and WooCommerce allows us to modify these template files. You can see these files in your WordPress installation directory as following path wp-content/plugins/woocommerce/templates/emails.
To use these template files, you have to override these email template files in your active theme. You have to make a folder “emails” in your theme and place the specific email template file that you want to override into it.
Override Template File
Let’s see a step-by-step guide on how to override WooCommerce email template files.
- Go to your active theme directory. I would recommend using a child theme for any customization to prevent the loss while updating themes or plugins.
- Create a ‘woocommerce‘ folder inside your theme folder.
- Create an ‘emails‘ folder inside ‘/woocommerce‘ folder.
- Now go to the plugins directory and find the ‘/woocommerce‘ plugin folder, inside the plugin, folder find the ‘/templates‘ folder and then the ‘/emails‘ folder. In this ‘/emails‘ folder, you will find all the email templates that woocommerce provides us by default.
- Select the template file that you want to modify and copy this file into your themes ‘woocommerce/emails‘ folder.
Following is the folder structure that you have to make to override the WooCommerce email template.
/plugins / woocommerce / templates / emails / email-header.php
to
/themes / {theme-name} / woocommerce / emails / email-header.php.
Let’s change the heading of the email or add the sub-heading of the email in the email-header.php file that you just copy into the themes directory.
In the email-header.php file, find this comment <!-- Header -->
and you will find the <h1>
heading tag inside it, so change it as you want or add a sub-heading along with the heading. See the following image for reference.
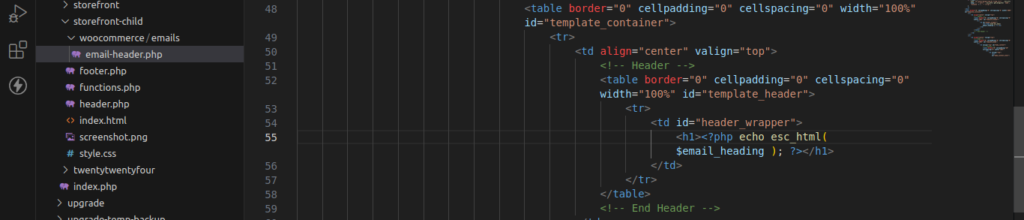
In this file, you can access the global variable $email_heading
and $email
and can add dynamic content based on conditions.
You can also change the layout of the HTML structure of these email template files by overriding them. But remember that some template files are common for all the emails, so be careful by changing the layout structure.
WooCommerce Custom Email Per Product
In addition to customizing email templates globally or conditionally, you may want to personalize emails based on the specific product purchased by the customer.
This level of customization can be achieved using the above hooks which allow you to modify the email and you can add specific information for specific products.
Also Read: Best Woocommerce Product Customizer Plugins
Let’s say you run an online store that sells a variety of products, including electronics, clothing, and home goods. Each product category has different post-purchase instructions or additional information that you want to communicate to your customers.
For example, when a customer purchases an electronic device like a smartphone, you might want to include instructions on how to set it up, activate warranties, or troubleshoot common issues. On the other hand, when a customer buys clothing, you may want to provide care instructions or styling tips. For home goods, you might want to include assembly instructions or safety guidelines.
To implement custom email templates per product in WooCommerce, you can utilize hooks and filters to dynamically modify the content of the order confirmation email based on the products purchased. See the below code.
Custom Email for Electronics Products
// Add custom email template for electronics products function custom_email_template_electronics( $order, $sent_to_admin, $plain_text, $email ) { // Check if the order contains electronics products $electronics_products = false; foreach ( $order->get_items() as $item ) { $product_id = $item->get_product_id(); $product = wc_get_product( $product_id ); if ( $product->is_type( 'electronics' ) ) { $electronics_products = true; break; } } // Load custom email template for electronics products if ( $electronics_products ) { // Load your custom email template file for electronics products wc_get_template( 'emails/custom-email-electronics.php', array( 'order' => $order ) ); } } add_action( 'woocommerce_email_order_details', 'custom_email_template_electronics', 10, 4 );
Custom Email for Clothing Products
// Add custom email template for clothing products function custom_email_template_clothing( $order, $sent_to_admin, $plain_text, $email ) { // Check if the order contains clothing products $clothing_products = false; foreach ( $order->get_items() as $item ) { $product_id = $item->get_product_id(); $product = wc_get_product( $product_id ); if ( $product->is_type( 'clothing' ) ) { $clothing_products = true; break; } } // Load custom email template for clothing products if ( $clothing_products ) { // Load your custom email template file for clothing products wc_get_template( 'emails/custom-email-clothing.php', array( 'order' => $order ) ); } } add_action( 'woocommerce_email_order_details', 'custom_email_template_clothing', 10, 4 );
You would need to create the custom email template files (custom-email-electronics.php
and custom-email-clothing.php
) in your theme or child theme’s WooCommerce templates directory and populate them with the desired content specific to electronics or clothing products.
Also Read: How to Create a Blog Post Template in WordPress?
Conclusion
Customizing WooCommerce order emails is a powerful way to enhance your brand’s identity, improve customer engagement, and provide a personalized shopping experience.
By understanding the importance of customization, familiarizing yourself with WooCommerce’s email system, and leveraging hooks for conditional customization, you can create email notifications that not only inform but also delight your customers.
Whether you’re updating the email header, tailoring content based on order status, or adding product-specific details, the possibilities for customization are endless, allowing you to stand out in the crowded inbox landscape.
Related Links
FAQs
To access WooCommerce email templates, navigate to your WordPress admin panel, then head to WooCommerce > Settings > Emails. Here, you can customize the subject, heading, content, and footer of each email notification.
Yes, you can use the woocommerce_email_header
hook to customize email headers based on specific criteria. For example, you can change the header of completed order emails to express gratitude using custom code.
Utilize the woocommerce_email_before_order_table
hook to inject custom content, such as promotional banners or introductory messages, before the order table in your WooCommerce emails.
You can use the woocommerce_order_item_meta_start
and woocommerce_order_item_meta_end
hooks to add or modify metadata for each item in the order. These hooks allow you to enhance the order item details with additional information.
You can use the woocommerce_email_footer
hook to add custom content to the email footer. This hook provides a convenient way to include branding elements, social media links, or legal disclaimers in your WooCommerce emails.